Python List: The Definitive Guide
List is one of the most frequently used containers in Python that lets you store many items in one place.

How to create a python list
You can create a list by wrapping values in square brackets []
:
ages = [10, 40, 20]
print(ages)
Output:
[10, 40, 20]
Here, we’ve got a list with three items: 10, 40, and 20.
Your list can have items of any type, not just numbers:
# mixed types in a single list
list_a = [1, "two", 3]
# list with repeated values
list_b = [1, 1, 2, 1]
# an empty list
list_c = []
The list constructor
The list
constructor lets you make a list without the square brackets from any existing sequence:
# an empty list
empty_list = list()
# a list of characters
char_list = list("abc")
print(char_list)
Output:
['a', 'b', 'c']
Let’s create a list of numbers with the range
function:
numbers = list(range(1, 10, 3))
print(numbers)
Output:
[1, 4, 7]
Getting an item from a list by its index
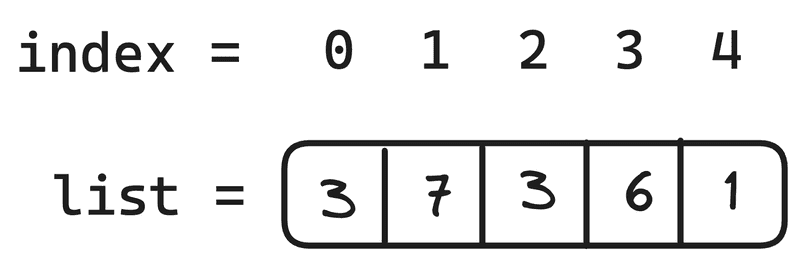
To grab an item from a list, just specify its number inside square brackets [<index>]
:
l = ["zero", "one", "two"]
item = l[2]
print(item)
Output:
two
how to change an item in a list
To change an item in the list, simply assign a new value to it by its index using the =
operator:
numbers = [1, 2, 3]
print('before:', numbers)
numbers[1] = 9
print('after:', numbers)
Output:
before: [1, 2, 3]
after: [1, 9, 3]
How to get the length of a list
Python has a function named len
to find out the length of a list. Just hand it a list, and it’ll tell you how many items are in there:
numbers = [1, 2, 3, 4]
print('len:', len(numbers))
Output:
len: 4
Negative index
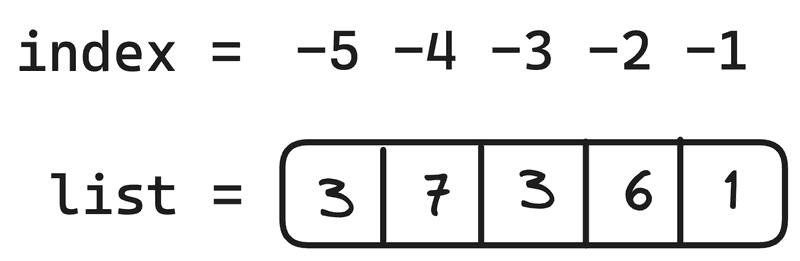
Python allows us to access items from the end using a negative index:
ages = [18, 24, 31]
print('last one: ', ages[-1])
print('second last:', ages[-2])
Output:
last one: 31
second last: 24
‘for’ loop with lists
Python lets us use the for
loop with lists. Just specify the list after the in
keyword:
students = ["John", "Kate", "Mike"]
for student in students:
print(student)
Output:
John
Kate
Mike
List of lists
You can have lists within lists to whatever depth you like:
matrix = [
[0, 1, 0],
[0, 2, 0],
[0, 0, 0],
]
# get an item
print("matrix[0][1] =", matrix[0][1])
# change an item
matrix[2][2] = 2
print(matrix)
Output:
matrix[0][1] = 1
[[0, 1, 0], [0, 2, 0], [0, 0, 2]]
Adding items to a list
We’ve seen how to make a list and tweak its individual items. But how do we add stuff to an existing list? The list
type offers a few neat methods for this.
list.append
The .append(item)
method lets you add an item at the end of a list:
l = [0, 1, 2]
l.append(3)
print(l)
Output:
[0, 1, 2, 3]
list.extend
If you wanna throw a bunch of items at the end all at once, go for the .extend(items)
method:
a = [0, 1, 2]
b = [3, 4]
a.extend(b)
print(a)
Output:
[0, 1, 2, 3, 4]
list.insert
When you’re need to add an item in the middle of a list, .insert(item)
is the way to go:
l = [3, 1, 0]
# shove 2 into index 1
l.insert(1, 2)
print(l)
Output:
[3, 2, 1, 0]
Removing an item from a list
Now that we’ve learned how to add items to an existing list, let’s learn how to remove them. There are a few methods provided by the list
type for this, but let’s start with the special keyword del
.
The del keyword
Python allows you to remove any item from a list using its index with the del
keyword:
l = [0, -3, 1]
del l[1]
print(l)
Output:
[0, 1]
list.remove
The .remove(value)
method removes the first occurrence of a value in the list:
l = [1, -3, 2, -3]
l.remove(-3)
print(l)
Output:
[1, 2, -3]
list.pop
The .pop(index=-1)
method removes the item at the specified position and returns its value:
l = [1, -3, 2, -4]
# by default, the last item is removed
print("pop:", l.pop(), " l:", l)
# here, we're removing the second item
print("pop:", l.pop(1), " l:", l)
Output:
pop: -4 l: [1, -3, 2]
pop: -3 l: [1, 2]
list.clear
The .clear()
method removes all items from the list:
l = [1, 2, 3]
l.clear()
print(l)
Output:
[]
Getting a slice of a list
Python offers an elegant way to fetch specific parts of a list. To get a slice, use the format [<start>:<end>:<step>]
.
The <end>
index item won’t be included in the slice. You can leave out the start, end, and step:
l = [0, 1, 2, 3, 4]
# here the slice is the entire list
print("l[:] =", l)
# here we're taking the 1st and 2nd items (end is exclusive)
print("l[1:3] =", l[1:3])
# here we're taking all items starting from the first
print("l[1:] =", l[1:])
# you can use negative indexes
# here we're grabbing all items starting from the second last
print("l[-2:] =", l[-2:])
# here we're taking items from the first to third, skipping every other
print("l[0:3:2] =", l[0:3:2])
# here the list is reversed
print("l[::-1] =", l[::-1])
Output:
l[:] = [0, 1, 2, 3, 4]
l[1:3] = [1, 2]
l[1:] = [1, 2, 3, 4]
l[-2:] = [3, 4]
l[0:3:2] = [0, 2]
l[::-1] = [4, 3, 2, 1, 0]
You can also use slices to modify lists:
l = [0, 1, 2, 3, 4]
print("original: ", l)
l[1:3] = [9, 8, 7]
print("modified: ", l)
l[1:4] = []
print("deleted: ", l)
Output:
original: [0, 1, 2, 3, 4]
modified: [0, 9, 8, 7, 3, 4]
deleted: [0, 3, 4]
Slices are a very handy tool when working with lists. I’d suggest getting comfortable working with them. It’ll greatly help you down the line.
Searching for values in a list
Now, let’s look at how you can search for items in a list.
The in keyword
Using value in [1, 2, 3]
will return True
if value
is in the list:
l = [1, 2, 3]
print("2 in l:", 2 in l)
print("5 in l:", 5 in l)
Output:
2 in l: True
5 in l: False
list.index
The .index(value)
method gives you the index of the first item that matches the given value. If the item isn’t found, index
will raise an error:
l = [1, 2, 3, 2]
print("l.index(2):", l.index(2))
print()
l.index(5) # <- will raise an error
Output:
l.index(2): 1
Traceback (most recent call last):
...
ValueError: 5 is not in list
If you only want to search within a part of the list, you can specify start
and end
arguments:
l = [1, 2, 3, 2, 5, 6]
# find the value 2 between the third and fifth items
print(l.index(2, 2, 4))
Output:
3
As you can see, the index
method returned the position of the second instance of the number 2.
list.count
The .count(value)
method can be used to count how many times a specific value appears in a list:
l = [1, 2, 3, 2, 3]
print(l.count(3))
Output:
2
sorting a list
If you need to sort a list, you can use the .sort()
method:
l = [3, 2, 4, 1]
l.sort()
print(l)
Output:
[1, 2, 3, 4]
Now, if you want to sort in descending order, you can add reverse=True
:
l = [3, 2, 4, 1]
l.sort(reverse=True)
print(l)
Output:
[4, 3, 2, 1]
You can also change the criteria for sorting:
l = ["one", "two", "ten"]
l.sort()
print("normal sort:", l)
# Here, we're sorting based on length
l.sort(key=len)
print("key sort: ", l)
Output:
normal sort: ['ten', 'two', 'one']
key sort: ['two', 'one', 'ten']
reversing a list
You already know that you can reverse a list using slices with a negative step:
l = [1, 2, 3]
print(l[::-1])
Output:
[3, 2, 1]
But in Python, you can also reverse a list without using slices. Just use the .reverse()
method:
l = [1, 2, 3]
l.reverse()
print(l)
Output:
[3, 2, 1]
making a list copy
Just like with reversing, you can use slices to make a copy:
a = [1, 2, 3]
b = a[:]
a[1] = 0
print("a:", a)
print("b:", b)
Output:
a: [1, 0, 3]
b: [1, 2, 3]
But if you’re not a fan of slices, you can use the .copy()
method:
a = [1, 2, 3]
b = a.copy()
a[1] = 0
print("a:", a)
print("b:", b)
Output is the same as before:
a: [1, 0, 3]
b: [1, 2, 3]
List comprehensions in Python
Python has a neat way to create lists based on certain conditions. These are called list comprehensions:
# Simple comprehension
print("1.", [x for x in range(0, 3)])
# Comprehension with filtering
print("2.", [x for x in range(0, 3) if x % 2 == 0])
# Comprehension with modification
print("3.", [x * 2 for x in range(0, 3)])
# Double loop comprehension
print("4.", [i * j for i in [1, 2] for j in range(1, 3)])
Output:
1. [0, 1, 2]
2. [0, 2]
3. [0, 2, 4]
4. [1, 2, 2, 4]
Unpacking lists
In Python, you can break down a list into individual values. To do this, just assign the list elements to variables:
a, b, c = [1, 2, 3]
print("sum:", a + b + c)
Output:
sum: 6
But, if the number of elements in the list doesn’t match the number of variables, you’ll get an error:
a, b = [1, 2, 3, 4, 5]
Error:
Traceback (most recent call last):
...
ValueError: too many values to unpack (expected 2)
Using the type function with lists
If you ever need to check whether a variable holds a list, you can use the type
function:
number = 1
l = [1, 2, 3]
print("Is number a list?:", type(number) == list)
print("Is l a list?: ", type(l) == list)
Output:
Is number a list?: False
Is l a list?: True
Adding lists together
In Python, you can merge two lists using the +
operator:
a = [1, 2, 3]
b = [4, 5, 6]
print(a + b)
Output:
[1, 2, 3, 4, 5, 6]
Multiplying lists
Sometimes, it’s handy to create a list where the values repeat. You can achieve this by multiplying a list by a number:
a = [1, 2]
b = a * 3
print(b)
Output:
[1, 2, 1, 2, 1, 2]
Comparing lists
If you have two lists and you want to compare them, you can use the standard ==
:
a = [1, 2, 3]
b = [1, 2, 3]
c = [3, 2, 1]
print("Is a equal to b?:", a == b)
print("Is b equal to c?:", b == c)
Output:
Is a equal to b?: True
Is b equal to c?: False
Exercises
- Creating and displaying a list:
Write a Python program that lets the user input data until they enter the word “stop”. All entered data should be stored in a list. After entering “stop”, the program should display the entire list. - Manipulating the list:
Write a program that asks the user to input 5 numbers. Store these numbers in a list and then display the highest, lowest, and the average of all the numbers in the list. - Operations with lists:
Create two lists: one with strings and another with numbers. Write a program that combines these lists into one and displays the result. Try using methods likeappend
andextend
, and see how they impact the outcome.
Discussion