srand in C++: initializing the random number generator
Hey there! In this article, we’re going to talk about the srand
function. This function is used in C++ to initialize the random number generator. We’ll start by understanding how this function works with examples, and then wrap up with some exercises for further practice.
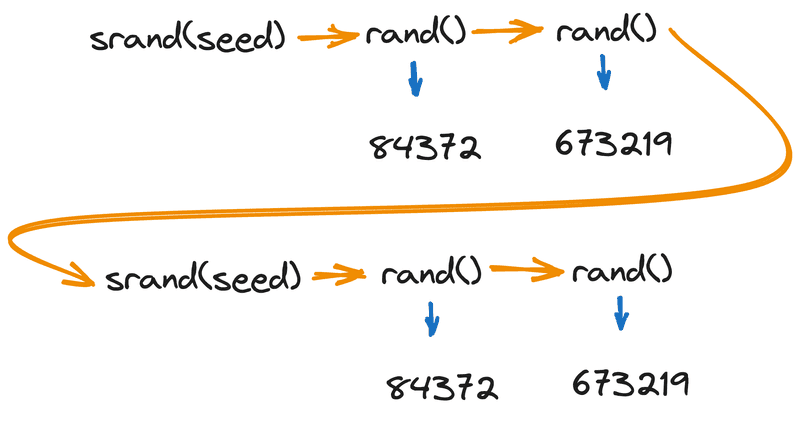
Initializing pseudo-random numbers with srand
The srand
function becomes available to us after including the header file <stdlib.h>
in C or <cstdlib>
in C++. The function signature looks like this:
void srand (unsigned int seed);
- The function takes a single argument, the seed, which will be used to calculate the sequence of random numbers.
- The function doesn’t return anything.
Let’s look at a simple example of using this function in tandem with rand
:
#include <cstdlib>
#include <iostream>
using namespace std;
int main() {
srand(123);
cout << "1. " << rand() << endl;
cout << "2. " << rand() << endl;
cout << "3. " << rand() << endl;
return 0;
}
Output:
1. 2067261
2. 384717275
3. 2017463455
Now, if we run this program again, the numbers will remain the same:
1. 2067261
2. 384717275
3. 2017463455
What will be displayed on the screen with the following code?
#include <cstdlib>
#include <iostream>
using namespace std;
int main() {
srand(456);
cout << "A. " << rand() << endl;
cout << "B. " << rand() << endl;
srand(456);
cout << "C. " << rand() << endl;
cout << "D. " << rand() << endl;
return 0;
}
To have different numbers each time, we need to change the seed for each run. Here are a few ways to achieve that:
- use the current time;
- ask the user to choose the seed at the start;
- use another random generator for initialization;
Using current time as the seed for random numbers
One of the most straightforward and convenient methods to initialize the random number generator is by using the current time. If you’re not running the program multiple times in a second, it’s good enough to use the time
function:
#include <cstdlib>
#include <ctime>
#include <iostream>
using namespace std;
int main() {
int seed = time(NULL);
srand(seed);
cout << "1. " << rand() << endl;
cout << "2. " << rand() << endl;
cout << "3. " << rand() << endl;
return 0;
}
Now, every time you run the program, you’ll get different random numbers. Here’s an example run:
1. 784904114
2. 2038884124
3. 128916889
And another one:
1. 786164639
2. 1749691329
3. 1568588132
As you can see, the numbers don’t repeat with every run.
User input as the seed
We can also achieve randomness by simply using the data the user inputs.
Let’s write a program as an example, which, based on the user’s age, predicts their salary. In this case, the result will be consistent for the same age, which is a significant advantage of this approach:
#include <cstdlib>
#include <iostream>
using namespace std;
int main() {
int age;
cout << "Enter your age: ";
cin >> age;
srand(age);
int salary = rand() % 100500 + 1;
cout << "Stars are saying your salary is: $" << salary << endl;
return 0;
}
Enter your age: 25
Your stellar salary: $18176
Using an External Random Number Generator
You don’t always have to rely on time or user data to initialize a random number generator. Leveraging system-generated random numbers is a good option. Although fetching these numbers takes longer compared to using rand
, the upside is that they’re almost always unique.
Let’s use the random_device
class from the <random>
header to obtain these system random numbers:
#include <cstdlib>
#include <iostream>
#include <random>
using namespace std;
int main() {
random_device system_rand;
int seed = system_rand();
srand(seed);
cout << "1. " << rand() << endl;
cout << "2. " << rand() << endl;
cout << "3. " << rand() << endl;
return 0;
}
Output:
1. 524804038
2. 666128437
3. 788388848
Every time you run this program, you’ll get different outputs. Plus, unlike when using the current time, you can run this program several times a second without recycling random numbers.
Exercises
-
Using
srand
with current time:
Write a program that initializessrand
using the current time as the seed. Your program should then generate and display five random numbers. Run it a few times and observe the results. -
Custom seed for
srand
:
Craft a program that asks the user for their favorite book, movie, or song title. Use the length of their input as the seed for thesrand
function. From this seed, generate and display three random numbers. -
Analyzing the behavior of
srand
:
Modify the program from the first exercise to display five random numbers, but this time let the user specify the seed. Run the program multiple times, first with the same seed and then with different ones. Analyze and describe what you observe.
Discussion