strcat in C/C++: string concatenation
Hey there! In this piece, I’m going to fill you in on what strcat
is and how to wield it. We’ll kick off with an example, then we’ll try our hand at crafting the function ourselves. We’ll wrap it all up with exercises for further practice.
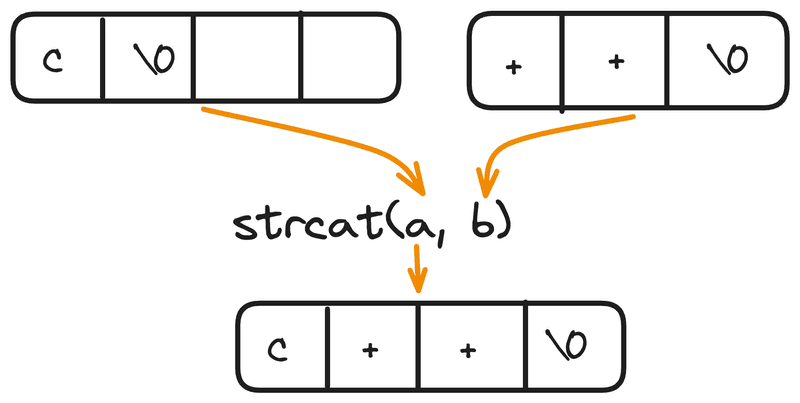
String concatenation using strcat in C/C++
Let’s dive right in and see how this function is structured:
char * strcat ( char * destination, const char * source );
- The first argument is the string to which we’ll be appending another string. It needs to be spacious enough to accommodate the result.
- The second argument is the string you’d like to append.
- The function then returns the first argument
destination
.
Both strings should terminate with a null character.
Oh, and before I forget, to use this function, you’ll need to include <cstring>
in C++ or <string.h>
in C.
Now, let’s see this function in action:
#include <cstring>
#include <iostream>
using namespace std;
int main() {
// the string needs to be roomy enough to house
// both strings after the merge
char destination[100] = "C";
// size isn't a big deal here
char plusplus[] = "++";
char* result = strcat(destination, plusplus);
cout << "plusplus:" << plusplus << endl;
cout << "result: " << result << endl;
cout << "destination: " << destination << endl;
cout << "result == destination: " << (result == destination) << endl;
return 0;
}
And let’s run this masterpiece:
plusplus:++
result: C++
destination: C++
result == destination: 1
Pretty straightforward, right? destination
mirrors result
. plusplus
remains untouched. And we’ve verified that result
and destination
point to the same string. All good.
What will be displayed on the screen?
#include <cstring>
#include <iostream>
using namespace std;
int main() {
char first_str[20] = "Open";
char second_str[] = "AI";
strcat(first_str, second_str);
cout << first_str << endl;
return 0;
}
Now, let’s stand out from the crowd by writing our own version of this function.
Crafting our own strcat, with blackjack and arrays
To demonstrate our coding skills, let’s create our own version of strcat
:
#include <cstring>
#include <iostream>
using namespace std;
char* my_strcat(char* destination, const char* source) {
int len = strlen(destination);
for (int i = 0; source[i] != '\0'; i++) {
destination[len + i] = source[i];
}
destination[len + strlen(source)] = '\0';
return destination;
}
int main() {
char result[100] = "ABC";
cout << "result = " << my_strcat(result, "DEF") << endl;
return 0;
}
Let’s run this thing:
result = ABCDEF
Phew! It worked without a hitch. Otherwise, I’d have been blushing big time.
You might have noticed I used the strlen
function. Here’s your chance to one-up me: try implementing my_strcat
without the help of built-in functions. Share your take in the comments.
Exercises
-
Basics of
strcat
usage:- Write a C++ program that employs
strcat
to merge two user-inputted strings. Ensure the resulting string matches the expected concatenation. - Modify the program to display all three strings (the original pair and the resultant combo).
- Write a C++ program that employs
-
Craft your own
strcat
:- Building on the example shared in the article, design your rendition of the
strcat
function. Call itmy_strcat
. - Test your function with a variety of string pairs and compare the outcomes to the native
strcat
function.
- Building on the example shared in the article, design your rendition of the
-
my_strcat
withoutstrlen
:- Try your hand at creating a version of
my_strcat
sans the built-instrlen
or any other pre-built functions. - Test your creation against different string pairs to ensure it’s up to snuff.
- Try your hand at creating a version of
Discussion