Python dictionaries (dict): A guide with examples
Dictionary (dict) plays an essential role in Python. Unlike list, dictionary stores data in a key -> value format.
In this article, we’ll dive deep into how to use this collection in your programs with illustrative examples.
What is a dictionary
A dictionary is a collection that stores items in a "key": "value"
format, also known as an associative array. It’s similar in use to lists, but there are some differences:
- Anything can be a key: from a simple string to complex objects.
- In newer versions of Python (>= 3.7), items are stored in the order they were added.
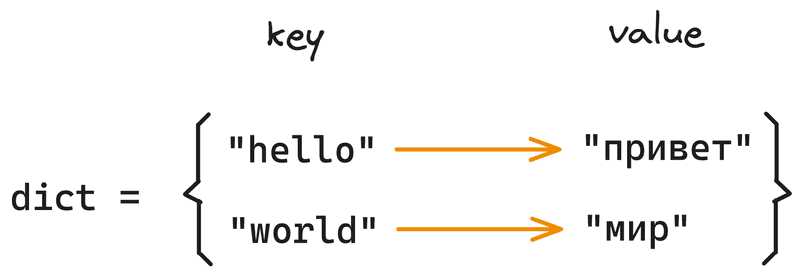
How to create a dictionary
To create a dictionary, we can use curly braces:
ages = {"Yura": 18, "Masha": 22, "Pasha": 30}
print(ages)
Output:
{'Yura': 18, 'Masha': 22, 'Pasha': 30}
There’s a second method: we can use the dict
constructor:
translations = dict(hello="hello", world="world")
print(translations)
Output:
{'hello': 'hello', 'world': 'world'}
In Python, you can also use the dict
constructor to create a dictionary from a list of key-value pairs:
translations = dict([
["hello", "hello"],
["world", "world"]
])
print(translations)
Output:
{'hello': 'hello', 'world': 'world'}
It’s worth noting that Python doesn’t allow having multiple items with the same key in a dictionary:
letters = {
"A": 1,
"A": 2,
}
print(letters)
Output:
{'A': 2}
As you can see, "A": 2
overwrote "A": 1
.
What will be printed on the screen?
dictionary = dict([
["apple", "apple"],
["orange", "orange"],
["apple", "apple-fruit"]
])
print(dictionary)
List of dictionaries
Since a dict
dictionary is just a value in Python, we can store dictionaries within other collections. For instance, we can create a list of dictionaries:
dictionaries = [
{"hello": "hello", "world": "world"},
{"A": 1, "B": 2, "C": 3},
]
print(dictionaries)
Output:
[{'hello': 'hello', 'world': 'world'}, {'A': 1, 'B': 2, 'C': 3}]
Fetching an item from the dictionary
To get the value of an item from a dictionary by its key, we can use square brackets just like with lists:
letters = {"A": 1, "B": 2, "C": 3}
print(letters["B"]) # 2
print(letters["C"]) # 3
However, if we try to access a non-existing key, we’ll get an error:
empty_dict = {}
print(empty_dict["no key"])
Error:
Traceback (most recent call last):
...
KeyError: 'no key'
To avoid an error when accessing a non-existing key, we can use the dict.get()
method:
names = {"Андрей": "Andrew", "Макс": "Max"}
print("Дима:", names.get("Дима"))
Output:
Дима: None
As you can see, the get()
method returned None
because there’s no “Дима” in the dictionary.
Dictionary length
To find out how many items are in a dictionary, just use the len()
function:
letters = {"A": 1, "B": 2, "C": 3}
print("number of letters:", len(letters))
The output you’ll get:
number of letters: 3
Adding an item to a dictionary
If you want to add a new item to a dictionary (or change an old one), just use the equal sign:
lang = {"C++": "static", "Rascal": "unknown"}
lang["Python"] = "dynamic"
lang["Rascal"] = "hybrid"
print(lang)
Here’s what you’ll see:
{'C++': 'static', 'Rascal': 'hybrid', 'Python': 'dynamic'}
What’s gonna show up on the screen?
fruits = {"apple": "red", "banana": "yellow"}
fruits["mango"] = "yellow"
fruits["banana"] = "green"
print(fruits)
If you’re in the mood to add or change multiple items at once, you can use the dict.update()
method:
lang = {"C++": "static", "Rascal": "unknown"}
lang.update(Python="dynamic", Rascal="hybrid")
print(lang)
Guess what? The output is the same as before:
{'C++': 'static', 'Rascal': 'hybrid', 'Python': 'dynamic'}
You can use another dictionary as an argument for the
update
method if you want.
There’s also this cool method that lets you add an item to a dictionary only if that key isn’t there already:
first_seen = {"Dima": "yesterday"}
first_seen.setdefault("Dima", "today")
first_seen.setdefault("Masha", "today")
print(first_seen)
Check it out:
{'Dima': 'yesterday', 'Masha': 'today'}
As you can see, setdefault
added “Masha” without messing with “Dima”.
Removing an item from a dictionary
Python’s got a bunch of ways to kick items out of a dictionary.
The easiest one? Just use the del
keyword:
population = {"Moscow": 12_000_000, "Abracadabra": 0}
del population["Abracadabra"]
print(population)
Here’s what you’ll get:
{'Moscow': 12000000}
But dictionaries also have this special pop
method you can use instead of del
:
population = {"Moscow": 12_000_000, "Abracadabra": 0}
value = population.pop("Abracadabra")
print(value)
print(population)
The output will look like:
0
{'Moscow': 12000000}
The pop()
method is pretty smart. If it can’t find a key, it’ll just return a default value:
empty = {}
print(empty.pop("key", "default value")) # output: default value
If you’re feeling like removing all items from a dictionary, just go with the dict.clear()
method:
letters = {"A": 1, "B": 2}
letters.clear()
print(letters)
Output:
{}
The dict.popitem()
method removes the last item you added to the dictionary:
letters = {"B": 2}
letters["A"] = 1
letter = letters.popitem()
print("kicked out:", letter)
print("leftovers:", letters)
Output:
kicked out: ('A', 1)
leftovers: {'B': 2}
Just a heads up, before Python 3.7,
popitem
would randomly pick an item to remove.
Iterating over dictionary elements
The for loop allows us to iterate through all the keys in a dictionary:
students = {"Kate": 20, "John": 30, "Mark": 40}
for name in students:
print(name, students[name])
Output:
Kate 20
John 30
Mark 40
dict.keys() method
The keys()
method provides all the keys stored in a dictionary:
students = {"Kate": 20, "John": 30, "Mark": 40}
for name in students.keys():
print(name)
Output:
Kate
John
Mark
By default, the for
loop iterates over the dictionary’s keys.
dict.values() method
To get values instead of keys, use the values()
method:
students = {"Kate": 20, "John": 30, "Mark": 40}
for age in students.values():
print(age)
Output:
20
30
40
dict.items() method
Often, it’s convenient to get both the key and its corresponding value when iterating over a dictionary. This is where the items()
method comes in handy:
students = {"Kate": 20, "John": 30, "Mark": 40}
for name, age in students.items():
print(name, age)
Output:
Kate 20
John 30
Mark 40
Dictionary within a dictionary
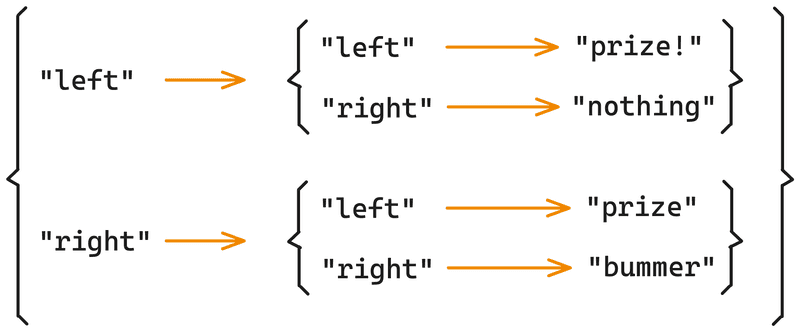
Since dictionaries in Python can hold any values, there’s nothing stopping us from creating a dictionary of dictionaries:
game = {
"left": {
"left": "empty",
"right": "prize!",
},
"right": {
"left": "empty",
"right": "consolation prize",
},
}
print(game["left"]["right"]) # prize!
print(game["right"]["right"]) # consolation prize
As you can see, working with nested dictionaries is no different than working with regular ones.
Checking for a key in a dictionary
Often, there’s a need to execute code depending on whether a particular key exists in the dictionary. The in
keyword lets you do just that:
country = {"name": "France", "population": 67_000_000}
if "name" in country:
print("Country:", country["name"])
else:
print("Nameless country")
Output:
Country: France
How to copy a dictionary
Python has a special method for copying dictionaries - copy()
:
original = {"A": 1, "B": 2}
copy = original.copy()
copy["C"] = 3
print("original:", original)
print("copy: ", copy)
Output:
original: {'A': 1, 'B': 2}
copy: {'A': 1, 'B': 2, 'C': 3}
If for some reason you don’t want to use the copy()
method, you can create a new dictionary using the dict
constructor:
original = {"A": 1, "B": 2}
copy = dict(original) # using the constructor instead of the copy method
copy["C"] = 3
print("original:", original)
print("copy: ", copy)
The output remains the same:
original: {'A': 1, 'B': 2}
copy: {'A': 1, 'B': 2, 'C': 3}
Dictionary comprehension
Python lets you create dictionaries from existing data using comprehension syntax:
sqr = {x: x * x for x in range(1, 4)}
print(sqr)
Output:
{1: 1, 2: 4, 3: 9}
Just like with list comprehension, you can throw in an if
to filter out certain dictionary elements:
sqr = {x: x * x for x in range(1, 8) if x % 2 == 0}
print(sqr)
Output:
{2: 4, 4: 16, 6: 36}
What’s the output of this Python code?
items = {"a": 1, "b": 2, "c": 3}
doubled = {k: v * 2 for k, v in items.items() if v % 2 != 0}
print(doubled)
Using the type() function for dictionaries
If you’re need to find out if a variable’s holding a dictionary, just use the type()
function:
number = 1
d = {1: 2, 2: 3}
print("Is number a dict?", type(number) == dict)
print("Is d a dict? ", type(d) == dict)
Output:
Is number a dict? False
Is d a dict? True
Exercises
- Creating a basic dictionary:
Write a Python program that lets users store key -> value pairs in a dictionary. Once the user’s done typing, show them the whole dictionary. - Key search:
Make a feature that lets users type in a key and the program displays the corresponding value from the dictionary. - Adding and removing elements:
Change your program so users can add new key -> value pairs or remove existing ones by their keys. Every time something changes, display the updated dictionary on the screen.
Discussion