strcmp in C/C++: compare two strings
👋 Hey there! In this article, we’re diving into the strcmp
function that allows you to compare two strings. We’ll start off with a few examples of how to use this function, then we’ll write our own version of it, and finally, there are exercises for further practice.
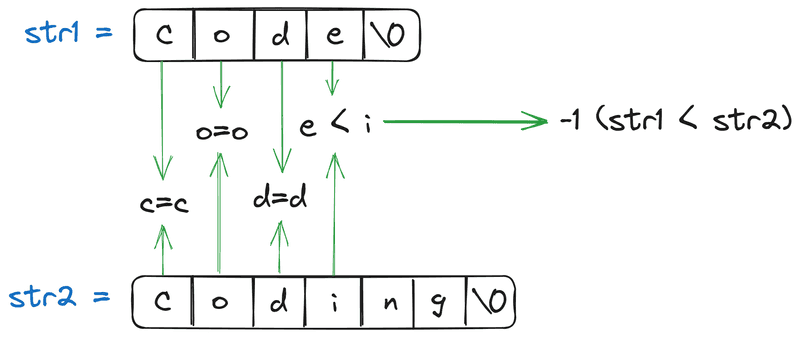
How to compare strings using strcmp
To compare two strings in C/C++, you can use the strcmp
function. This function becomes available after you include the <string.h>
file. In C++, you can include <cstring>
.
strcmp
takes two strings and compares each character in sequence one by one. Once it finds the first differing character, it returns:
1
if the character in the first string is greater than the one in the second.-1
if this first differing character is lesser in the first string.0
if the strings are equal (no differing characters).
If it doesn’t find any differing characters but one string ends before the other, that string is considered the lesser of the two. We’ll see how this works with an example.
The prototype of this function looks like:
int strcmp ( const char * a, const char * b );
- The first argument should be a pointer to a C-style string (C-style strings end with a
'\0'
character). - The second argument should be a pointer to the string you want to compare with.
- The function returns
1
,-1
, or0
depending on which string is “greater”.
Let’s see how this function works with an example:
#include <stdio.h>
#include <string.h>
void compare(const char* a, const char* b) {
printf("strcmp(\"%s\", \"%s\") = %d\n", a, b, strcmp(a, b));
}
int main() {
compare("abc", "abc");
compare("abc", "ab");
compare("ab", "abc");
compare("abc", "bcd");
compare("bc", "abc");
return 0;
}
Program output:
strcmp("abc", "abc") = 0
strcmp("abc", "ab") = 1
strcmp("ab", "abc") = -1
strcmp("abc", "bcd") = -1
strcmp("bc", "abc") = 1
What will the output of the following code be?
#include <stdio.h>
#include <string.h>
int main() {
char str1[] = "apple";
char str2[] = "application";
printf("%d", strcmp(str1, str2));
return 0;
}
Implementing your own strcmp
Let’s craft our own version of the strcmp
function without using any built-in libraries to get a better grip on its inner workings:
#include <stdio.h>
#include <string.h>
int my_strcmp(const char* a, const char* b) {
int result = 0;
// search for the first differing character until we reach the end of one of the strings
// we don't need to check *b != '\0` since the first condition for the loop to continue
// is that *a equals *b. If *a equals *b and *a isn't '\0`, that means *b isn't '\0` either
while (*a == *b && *a != '\0') {
a++;
b++;
}
// if both strings have ended, they are equal
if (*a == '\0' && *b == '\0') {
return 0;
}
// here, we compare the first differing character
if (*a > *b) {
return 1;
} else {
return -1;
}
}
void compare(const char* a, const char* b) {
printf("my_strcmp(\"%s\", \"%s\") = %d\n", a, b, my_strcmp(a, b));
}
int main() {
compare("abc", "abc");
compare("abc", "ab");
compare("ab", "abc");
compare("abc", "bcd");
compare("bc", "abc");
return 0;
}
Program output:
my_strcmp("abc", "abc") = 0
my_strcmp("abc", "ab") = 1
my_strcmp("ab", "abc") = -1
my_strcmp("abc", "bcd") = -1
my_strcmp("bc", "abc") = 1
As you can see, our function works just like the standard library’s strcmp
function.
Exercises
-
Comparing Strings Using
strcmp
:
Write a C program that prompts the user to input two strings and compares them using thestrcmp
function. The program should display the comparison result in a user-friendly format. Test your program with various string pairs. -
Implement Your Own Version of
strcmp
:
Based on the example in the article, craft your version of thestrcmp
function. Then, validate its effectiveness by comparing the results with the built-instrcmp
function on various examples. -
Extending the Functionality:
Enhance your program by not just comparing the strings, but also determining the length of each string before the comparison. Display the length of each string before showing the comparison result.
Discussion