sqrt in C/C++: calculating square root
👋 Hey! In this article, we will discuss the sqrt
function, which is used to compute the square root of a number in C/C++. We will start with a few examples, and then proceed to create our own implementation. Additionally, we will explore the differences between sqrt
, sqrtl
, and sqrtf
. At the end, there will be exercises to strengthen your understanding of the material.
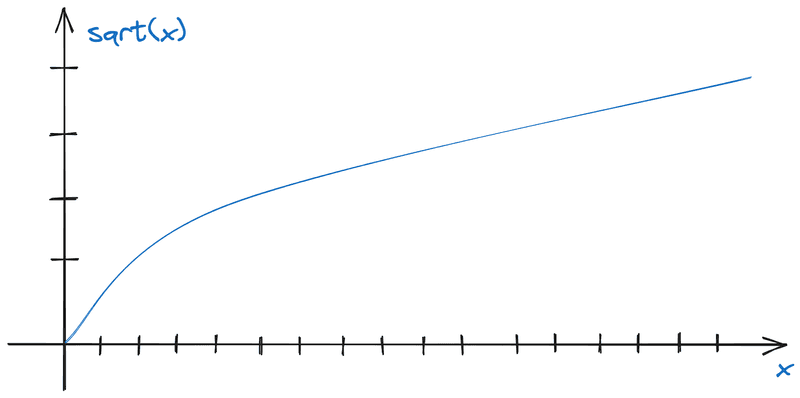
Calculating the square root of a number in C/C++
The built-in sqrt
function in C/C++ is used to compute the square root of a number. To access this function, you need to include the <math.h>
header file in C or <cmath>
in C++. The prototype of the function is as follows:
double sqrt (double x);
- The function accepts a single argument,
x
, which is the number for which we want to compute the square root. - It returns the square root of
x
.
Let’s look at an example:
#include <cmath>
#include <iostream>
using namespace std;
int main() {
cout << "sqrt(4) = " << sqrt(4) << endl;
cout << "sqrt(1024) = " << sqrt(1024) << endl;
cout << "sqrt(2) = " << sqrt(2) << endl;
cout << "sqrt(0) = " << sqrt(0) << endl;
cout << "sqrt(0.25) = " << sqrt(0.25) << endl;
cout << "sqrt(-2) = " << sqrt(-2) << endl;
return 0;
}
Output of the program:
sqrt(4) = 2
sqrt(1024) = 32
sqrt(2) = 1.41421
sqrt(0) = 0
sqrt(0.25) = 0.5
sqrt(-2) = nan
Notice that the sqrt
function returns nan
for negative numbers because it is mathematically impossible to obtain a real square root of a negative number. This behavior should be considered when developing your programs.
What will be displayed on the screen?
#include <iostream>
#include <cmath>
using namespace std;
int main() {
double a = -49;
cout << sqrt(a) << endl;
return 0;
}
Implementing sqrt function yourself
Now, let’s create our own implementation of the sqrt
function using the binary search algorithm:
#include <cmath>
#include <iostream>
using namespace std;
double my_sqrt(double x) {
// cannot calculate the root of a negative number
if (x < 0) {
return NAN;
}
// search from zero to x + 1
// if x is less than one, the root will be larger than x, hence + 1
double left = 0, right = x + 1;
// adjust the number of iterations for different precision
for (int i = 0; i < 20; i++) {
double middle = (left + right) / 2;
if (middle * middle < x) {
left = middle;
} else {
right = middle;
}
}
return left;
}
int main() {
cout << "my_sqrt(4) = " << my_sqrt(4) << endl;
cout << "my_sqrt(1024) = " << my_sqrt(1024) << endl;
cout << "my_sqrt(2) = " << my_sqrt(2) << endl;
cout << "my_sqrt(0) = " << my_sqrt(0) << endl;
cout << "my_sqrt(0.25) = " << my_sqrt(0.25) << endl;
cout << "my_sqrt(-2) = " << my_sqrt(-2) << endl;
return 0;
}
Output:
my_sqrt(4) = 2
my_sqrt(1024) = 32
my_sqrt(2) = 1.41421
my_sqrt(0) = 0
my_sqrt(0.25) = 0.5
my_sqrt(-2) = nan
In the program above, we perform twenty iterations of the search. Adjusting the number of iterations will affect the precision of the result.
sqrtl and sqrtf functions
The sqrtl
and sqrtf
functions, similar to sqrt
, compute the square root of a number. However, they work with numbers of types long double
(sqrtl
) and float
(sqrtf
). Here’s an example:
#include <cmath>
#include <iostream>
using namespace std;
int main() {
cout << "sqrtl(1234) = " << sqrtl(1234) << endl;
cout << "sqrt (1234) = " << sqrt(1234) << endl;
cout << "sqrtf(1234) = " << sqrtf(1234) << endl;
return 0;
}
Output:
sqrtl(1234) = 35.1283
sqrt (1234) = 35.1283
sqrtf(1234) = 35.1283
As you can see, all three functions return the same result, but of different types.
Exercises
-
Using
sqrt
:
Write a C++ program that prompts the user for a floating-point number, applies thesqrt
function, and displays the result along with the original number entered by the user. -
Implementing your own
sqrt
forfloat
:
Modify the example provided in the article to create your own version of thesqrt
function that works withfloat
numbers. Test its functionality with various numbers. -
Comparing
sqrt
,sqrtf
, andsqrtl
:
Develop a program that illustrates the differences betweensqrt
,sqrtf
, andsqrtl
. The program should use all three functions, and display their results on the screen.
Discussion