strlen in C/C++: string length
👋 Hey there! In this article, we’re going to talk about the strlen
function. This function calculates the number of characters in a C-string. We’ll start off with a few examples of how it’s used, then we’ll roll up our sleeves and implement it ourselves, and by the end, you’ll find some cool exercises to seal the deal.
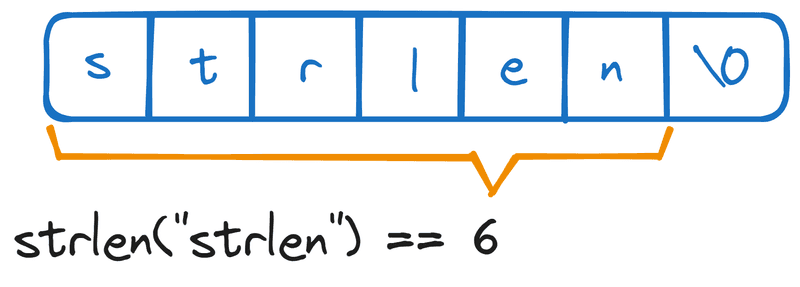
How to get the character count in C/C++
In C, if you want to get the character count of a string, you can make use of the strlen
function. This function becomes available once you include the <string.h>
header file. The prototype of this function looks like this:
size_t strlen ( const char * str );
- The sole argument this function accepts is a pointer to a null-terminated string (an array of characters that ends with the special character
'\0'
). - The function returns the count of characters in the string, excluding the
'\0'
.
Let’s dive into an example:
#include <stdio.h>
#include <string.h>
int main() {
char str[64] = "strlen in C";
int n = strlen(str);
printf("n = %d", n);
return 0;
}
Output:
n = 11
As you can see, strlen
returned the number of characters stored in the str
string.
Remember, strlen
doesn’t consider the size of the array holding the string. It merely counts the bytes from the string’s beginning up to the '\0'
. Here’s another example to illustrate this:
#include <stdio.h>
#include <string.h>
int main() {
char str[] = {'1', '2', '3', '\0', '4', '5'};
int n = strlen(str);
printf("n = %d", n);
return 0;
}
Output:
n = 3
In this case, the program outputs 3
, ignoring the characters '4'
and '5'
that come after the '\0'
.
What will be displayed on the screen?
#include <stdio.h>
#include <string.h>
int main() {
char str1[] = {'H', 'e', 'l', 'l', 'o', '\0', ' ', 'W', 'o', 'r', 'l', 'd', '!', '\0'};
char str2[] = "Hello";
int result = strlen(str1) + strlen(str2);
printf("%d", result);
return 0;
}
Crafting your own strlen
Now, let’s try writing this function ourselves. It’ll help us understand its inner workings. Our implementation will go through the string until we encounter the '\0'
:
#include <stdio.h>
int my_strlen(const char* s) {
int result = 0;
while (*s != '\0') {
s++;
result++;
}
return result;
}
int main() {
char str[] = "string in C";
int n = my_strlen(str);
printf("n = %d", n);
return 0;
}
Output of the program:
n = 11
Unsurprisingly, our version of the strlen
function behaves just like the built-in one.
Exercises
-
Using
strlen
:
Write a C program that prompts the user for a string, and using thestrlen
function, determines and displays the length of that string. The program should also display the string entered by the user. -
Building Your Own
strlen
:
Based on the example shared in this article, create your version of thestrlen
function and test it with different strings. Compare the results of your function with the standardstrlen
function. -
Handling Multiple Null-Terminated Characters:
Craft a program that creates a string containing several'\0'
characters within it, showcasing the differences (or lack thereof) in character counting between yourstrlen
version and the standardstrlen
function.
Discussion